- GuruFinance Insights
- Posts
- How To Use Python For Stocks Trading
How To Use Python For Stocks Trading
Understanding Moving Averages in Trading - Part 1
From Wall Street to Your Portfolio: Master Value Investing
The Applied Value Investing Certificate Program from Wharton Online and Wall Street Prep is an 8-week, online, self-paced program that teaches participants how to identify undervalued stocks with the process-driven approach used by the world’s top investors.
Program benefits also include:
Guest Speaker Series with top industry professionals
Exclusive access to networking and recruitment events
Invitation-Only LinkedIn Groups and Slack Channels
Certificate issued by Wharton Online and Wall Street Prep
Exciting News: Paid Subscriptions Have Launched! 🚀
On September 1, we officially rolled out our new paid subscription plans at GuruFinance Insights, offering you the chance to take your investing journey to the next level! Whether you're just starting or are a seasoned trader, these plans are packed with exclusive trading strategies, in-depth research paper analysis, ad-free content, monthly AMAsessions, coding tutorials for automating trading strategies, and much more.
Our three tailored plans—Starter Investor, Pro Trader, and Elite Investor—provide a range of valuable tools and personalized support to suit different needs and goals. Don’t miss this opportunity to get real-time trade alerts, access to masterclasses, one-on-one strategy consultations, and be part of our private community group. Click here to explore the plans and see how becoming a premium member can elevate your investment strategy!
Check Out Latest Premium Articles
The Moving Average (MA) is a widely used tool in technical analysis, valued by traders for its ability to streamline price data over a given timeframe.
The main function of the MA is to eliminate random price volatility, enabling traders to concentrate on the broader trend direction.

Source
Uptrend: When the price remains steadily above the moving average, it often indicates a bullish market or an upward trend.
Downtrend: Conversely, when the price stays consistently below the moving average, it generally suggests a bearish market or a downward trend.
We'll use Tesla's stock data to illustrate these concepts.import yfinance as yf
import mplfinance as mpf
df = yf.download('TSLA', start='2023-01-01', end='2024-01-01')
df.columns = df.columns.get_level_values(0)
mpf.plot(df, type='candle', style='charles', title='TSLA Candlestick Chart', volume=True)

TSLA Candlestick Chart. Image by author.
Simple Moving Average (SMA)
It is the unweighted average of prices over a specific number of periods.
import matplotlib.pyplot as plt
# Calculate the Simple Moving Averages (SMA) for different periods
df['SMA_20'] = df['Close'].rolling(window=20).mean()
df['SMA_50'] = df['Close'].rolling(window=50).mean()
df['SMA_100'] = df['Close'].rolling(window=100).mean()
# Plot the closing price and multiple SMAs using mplfinance
plt.figure(figsize=(12, 8))
# Plot closing price
plt.plot(df.index, df['Close'], label='Close Price', alpha=0.5)
# Plot 20-day, 50-day, and 100-day SMAs
plt.plot(df.index, df['SMA_20'], label='20-day SMA', alpha=0.8, color='orange')
plt.plot(df.index, df['SMA_50'], label='50-day SMA', alpha=0.8, color='green')
plt.plot(df.index, df['SMA_100'], label='100-day SMA', alpha=0.8, color='red')
# Customize the plot
plt.title('Tesla Stock Price with Multiple SMAs (20, 50, 100 days)')
plt.xlabel('Date')
plt.ylabel('Price (USD)')
plt.legend()
# Display the plot
plt.show()

Different SMAs. Image by author.
Exponential Moving Average (EMA)
It gives more weight to recent prices, making it more responsive to price changes compared to the SMA.
# Calculate the Exponential Moving Averages (EMA) for different periods
df['EMA_20'] = df['Close'].ewm(span=20, adjust=False).mean()
df['EMA_50'] = df['Close'].ewm(span=50, adjust=False).mean()
df['EMA_100'] = df['Close'].ewm(span=100, adjust=False).mean()
# Plot the closing price and multiple EMAs using matplotlib
plt.figure(figsize=(12, 8))
# Plot closing price
plt.plot(df.index, df['Close'], label='Close Price', alpha=0.5)
# Plot 20-day, 50-day, and 100-day EMAs
plt.plot(df.index, df['EMA_20'], label='20-day EMA', alpha=0.8, color='orange')
plt.plot(df.index, df['EMA_50'], label='50-day EMA', alpha=0.8, color='green')
plt.plot(df.index, df['EMA_100'], label='100-day EMA', alpha=0.8, color='red')
# Customize the plot
plt.title('Tesla Stock Price with Multiple EMAs (20, 50, 100 days)')
plt.xlabel('Date')
plt.ylabel('Price (USD)')
plt.legend()
# Display the plot
plt.show()
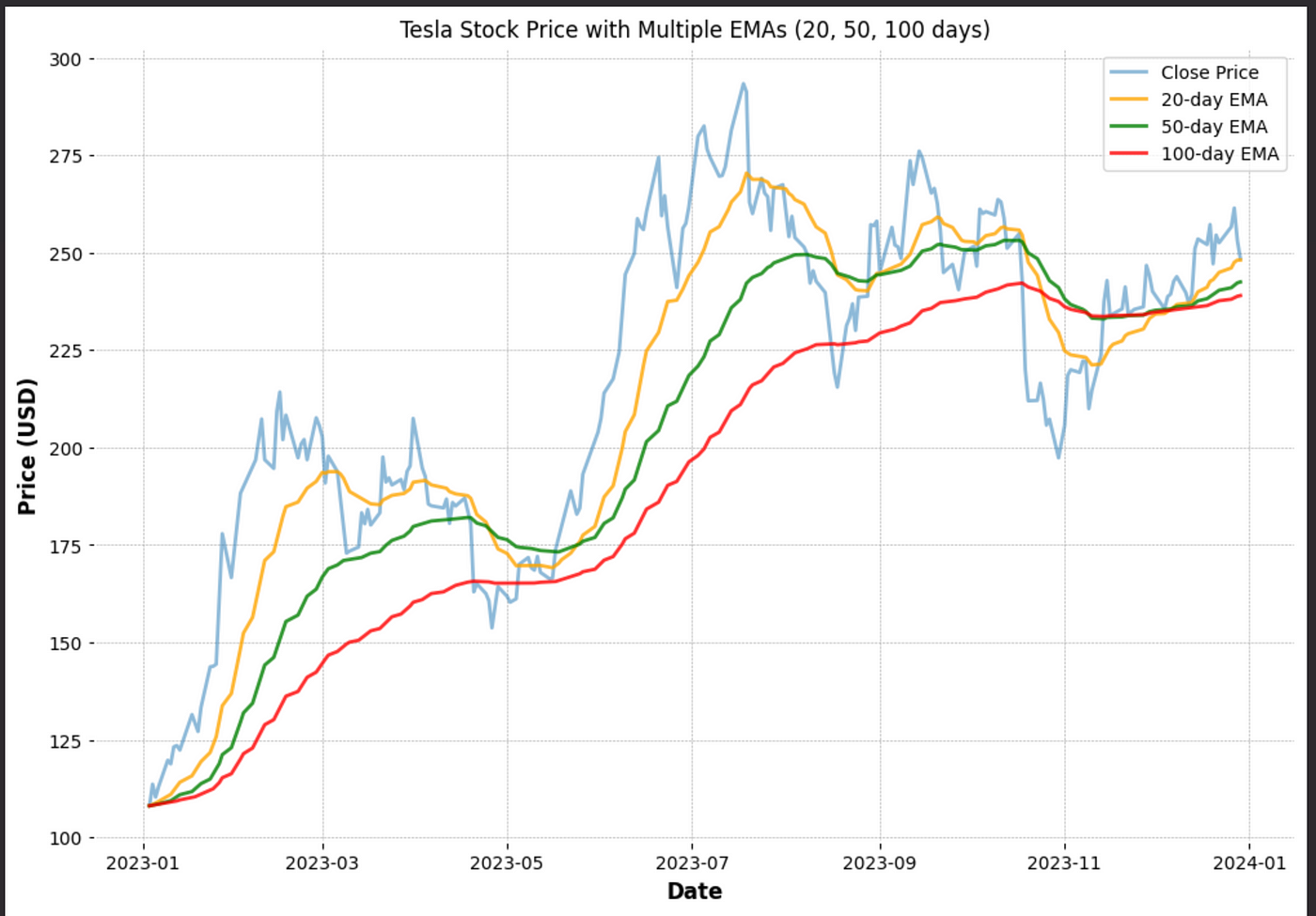
Multiple EMAs. Image by author.
Signals
Bullish Signal: If the price is above the moving average, it suggests a bullish trend.
Bearish Signal: If the price is below the moving average, it suggests a bearish trend.

Bullish vs Bearish. Source
Crossover Strategy
Golden Cross: A bullish signal occurs when a short-term MA (e.g., 20-day) crosses above a long-term MA (e.g., 50-day).
Death Cross: A bearish signal occurs when a short-term MA crosses below a long-term MA.

Golden Cross. Source

Death Cross. Source
# Calculate the 20-day and 50-day Simple Moving Averages (SMA)
df['SMA_20'] = df['Close'].rolling(window=20).mean()
df['SMA_50'] = df['Close'].rolling(window=50).mean()
# Identify Golden Cross and Death Cross
df['Golden_Cross'] = (df['SMA_20'] > df['SMA_50']) & (df['SMA_20'].shift(1) <= df['SMA_50'].shift(1))
df['Death_Cross'] = (df['SMA_20'] < df['SMA_50']) & (df['SMA_20'].shift(1) >= df['SMA_50'].shift(1))
# Plot the closing price, 20-day SMA, and 50-day SMA
plt.figure(figsize=(12, 8))
# Plot closing price
plt.plot(df.index, df['Close'], label='Close Price', alpha=0.5)
# Plot the SMAs
plt.plot(df.index, df['SMA_20'], label='20-day SMA', color='orange', alpha=0.8)
plt.plot(df.index, df['SMA_50'], label='50-day SMA', color='blue', alpha=0.8)
# Mark Golden Cross (bullish) points
plt.plot(df.index[df['Golden_Cross']], df['SMA_20'][df['Golden_Cross']], '^', markersize=10, color='green', lw=0, label='Golden Cross')
# Mark Death Cross (bearish) points
plt.plot(df.index[df['Death_Cross']], df['SMA_20'][df['Death_Cross']], 'v', markersize=10, color='red', lw=0, label='Death Cross')
# Customize the plot
plt.title('Tesla Stock Price with Golden and Death Crosses (20-day and 50-day SMA)')
plt.xlabel('Date')
plt.ylabel('Price (USD)')
plt.legend()
# Display the plot
plt.show()

Golden and Death Crosses. Image by author.
Support & Resistance
Support: In an uptrend, the moving average can act as a floor, preventing the price from falling.
Resistance: In a downtrend, the moving average can act as a ceiling, preventing the price from rising.

Support & Resistance. Source
Limitations
Lagging Indicator: Moving averages are lagging indicators, meaning they are based on past prices and may not respond quickly to sudden market changes.
Whipsaws: In volatile markets, moving averages can generate false signals or “whipsaws” (frequent crossing of price and the MA without a clear trend).
Common Time Perios
Short-term: 5, 10, 20 periods — used for short-term trends and signals.
Medium-term: 50 periods — popular for observing intermediate trends.
Long-term: 100, 200 periods — used for long-term trend analysis.
The insights and visual tools shared in this blog post, including the application of moving averages to identify support, resistance, and trading signals (such as the Golden Cross and Death Cross), are intended solely for educational purposes. Markets like stocks and cryptocurrencies are highly volatile and subject to rapid, unpredictable changes. Past performance using these techniques does not ensure future success. Always conduct in-depth research, consider seeking advice from a financial professional, and fully understand the risks before making trading decisions. Apply these strategies responsibly and with care.