- GuruFinance Insights
- Posts
- Unlocking Stock Trading Insights: Using the Volume Ratio for Smarter Decision-Making with Python”
Unlocking Stock Trading Insights: Using the Volume Ratio for Smarter Decision-Making with Python”
Leveraging Volume Ratios to Identify Market Momentum and Predict Price Movements in Stock Trading
Dive into the World of Value Investing with Wharton Online
The Applied Value Investing Certificate program provides a comprehensive 8-week online experience for those looking to implement the same value investing strategies and approaches used by the world’s top investors.
Beyond mastering technical skills from top Wall Street instructors, you'll gain insights from industry leaders like Jeff Gramm, author of The Education of Value Investor, and Howard Marks, Co-Founder of Oaktree Capital.
Plus, enjoy exclusive networking opportunities, LinkedIn groups, and targeted recruitment events with leading firms.
Exciting News: Paid Subscriptions Have Launched! 🚀
On September 1, we officially rolled out our new paid subscription plans at GuruFinance Insights, offering you the chance to take your investing journey to the next level! Whether you're just starting or are a seasoned trader, these plans are packed with exclusive trading strategies, in-depth research paper analysis, ad-free content, monthly AMAsessions, coding tutorials for automating trading strategies, and much more.
Our three tailored plans—Starter Investor, Pro Trader, and Elite Investor—provide a range of valuable tools and personalized support to suit different needs and goals. Don’t miss this opportunity to get real-time trade alerts, access to masterclasses, one-on-one strategy consultations, and be part of our private community group. Click here to explore the plans and see how becoming a premium member can elevate your investment strategy!
Check Out Latest Premium Articles
The stock market can be quite complex, but certain tools and indicators can help simplify the analysis. One such useful tool is the ‘Volume Ratio’ — a powerful metric for identifying irregular volume activity. This ratio gives valuable insights into a stock’s momentum and, when combined with directional indicators, can strengthen the prediction of future price movements.
In this article, we’ll explore why volume plays such a critical role in stock trading, how the volume ratio adds significance to the analysis, and how you can leverage Python to apply this metric effectively. By the end, you’ll be equipped with a handy Python script that enhances your ability to utilize the volume ratio, empowering you to make more informed trading decisions. Below is an example of the analytical output we’ll walk through in this guide.
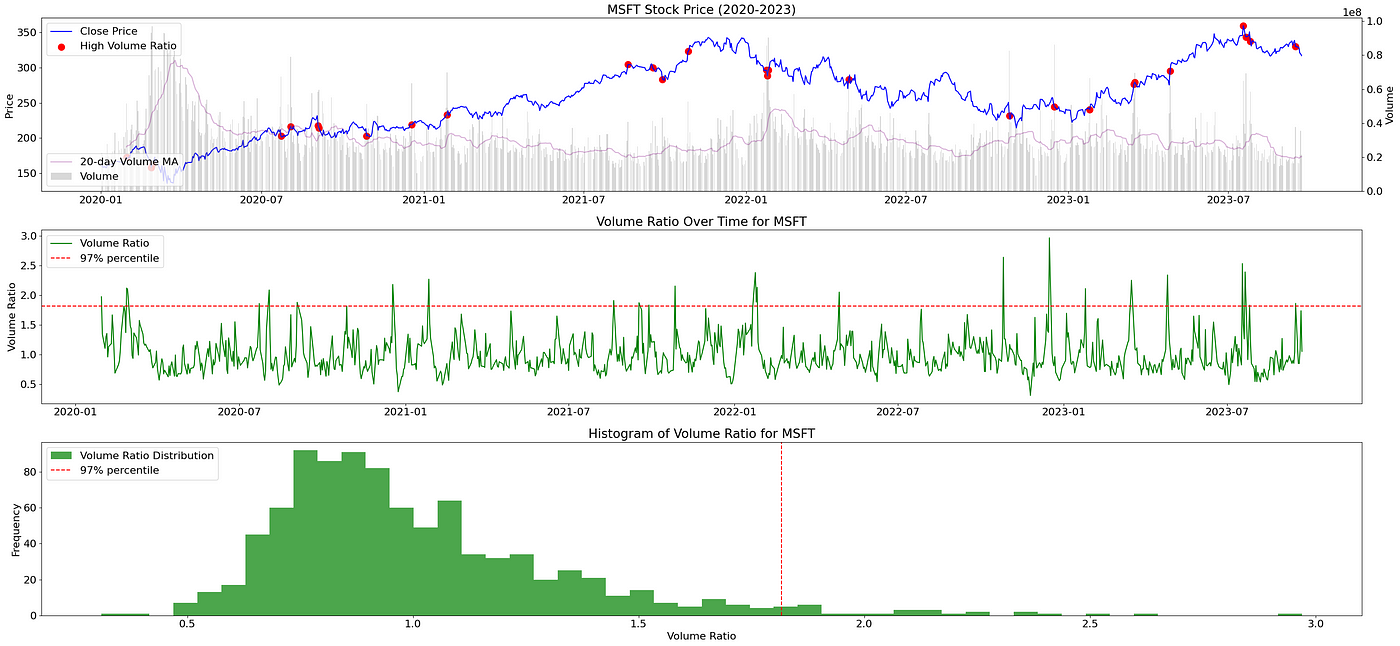
1. The Importance of Trading Volume
Hands Down Some Of The Best 0% Interest Credit Cards
Pay no interest until nearly 2027 with some of the best hand-picked credit cards this year. They are perfect for anyone looking to pay down their debt, and not add to it!
Click here to see what all of the hype is about.
1.1 Volume in Stock Market Analysis
Volume represents the total number of shares traded within a specific timeframe, and it serves as a key metric for assessing market dynamics. High trading volume often reflects strong market interest and participation, while shifts or irregularities in volume could indicate noteworthy changes or events warranting further exploration.
In trading analysis, volume is a fundamental indicator of a stock’s momentum. For instance, a significant price change on low volume often points to weak market consensus behind the move, whereas a price shift accompanied by high volume tends to suggest a stronger, potentially longer-lasting market trend.
1.2 The Role of the Volume Ratio
While observing raw volume numbers can offer valuable insights, it’s important to assess these figures in context. That’s where the Volume Ratio comes into play, standardizing volume data for more accurate analysis.
The Volume Ratio compares the current trading volume to its average over a recent period, allowing traders to measure shifts in stock momentum. When the Volume Ratio spikes above 1, it suggests that current volume is significantly higher than the historical average for that timeframe. These spikes often signify external catalysts that may lead to notable price movements in either direction.
Thus, volume serves as a barometer for trading activity, while the Volume Ratio identifies patterns and outliers in this activity, helping traders detect potential opportunities, particularly when volume deviates from the norm.
2. Python Implementation
For implementing the Volume Ratio, we will utilize three key Python libraries:
pandas: Used for managing and analyzing data sets.
matplotlib: Used to create visual representations of the data.
yfinance: This library allows us to easily fetch historical and current stock data from Yahoo Finance.
Fetching and Processing the Data:
Our analysis will focus on Microsoft (MSFT), analyzing stock data between January 1, 2020, and December 22, 2023.
# Define the stock ticker symbol for Microsoft
stock_ticker = 'MSFT'
# Set the percentile threshold to capture top 3% of high-volume trading days
threshold_percentile = 0.97
# Download historical stock data for Microsoft from 2020 to December 2023
historical_data = yf.download(stock_ticker, start='2020-01-01', end='2023-12-22')
Next, we’ll calculate the 50-day and 200-day moving averages for the Close price to provide further insights. After that, we’ll compute the 20-day moving average for the volume, followed by the Volume Ratio, which will serve as a critical metric for understanding trading dynamics.
data['50_day_MA'] = data['Close'].rolling(window=50).mean() # Calculate the 50-day moving average for closing price
data['200_day_MA'] = data['Close'].rolling(window=200).mean() # Calculate the 200-day moving average for closing price
data['Volume_MA20'] = data['Volume'].rolling(window=20).mean() # Calculate the 20-day moving average for volume
data['Volume_Ratio'] = data['Volume'] / data['Volume_MA20'] # Compute the ratio of current volume to the 20-day moving average volume
Visualization Overview:
In this section, we will illustrate the stock dynamics of Microsoft through a comprehensive visualization. We’ll depict the stock’s closing price, the trajectory of its volume ratio over time, and provide a histogram showcasing the distribution of the volume ratio values.
The primary chart will focus on Microsoft’s stock price, highlighting days where the volume ratio exceeds the set threshold, indicating periods of significant trading activity. The secondary charts will offer insight into the changes in the volume ratio over time and how it is distributed, with particular attention to instances where the ratio surpasses the chosen threshold.
import pandas as pd
import matplotlib.pyplot as plt
import yfinance as yf
# Set up the stock symbol and threshold percentile
stock_symbol = 'MSFT'
percentile_level = 0.97
# Retrieve historical data
stock_data = yf.download(stock_symbol, start='2020-01-01', end='2023-12-22')
# Calculate moving averages
stock_data['Short_Term_MA'] = stock_data['Close'].rolling(window=50).mean()
stock_data['Long_Term_MA'] = stock_data['Close'].rolling(window=200).mean()
# Compute volume moving average and ratio
stock_data['Avg_Volume_20'] = stock_data['Volume'].rolling(window=20).mean()
stock_data['Volume_Index'] = stock_data['Volume'] / stock_data['Avg_Volume_20']
# Determine dynamic threshold based on volume ratio
dynamic_threshold = stock_data['Volume_Index'].quantile(percentile_level)
highlight_mask = stock_data['Volume_Index'] > dynamic_threshold
# Prepare the figure for subplots
fig, (price_ax, volume_ax, hist_ax) = plt.subplots(3, figsize=(30, 14))
# Plot closing price in price_ax
price_ax.plot(stock_data.index, stock_data['Close'], label='Closing Price', color='blue')
price_ax.scatter(stock_data.index[highlight_mask], stock_data['Close'][highlight_mask], color='red', s=100, label='High Volume Days')
price_ax.set_title(f'{stock_symbol} Price Movement (2020–2023)')
price_ax.set_ylabel('Price')
price_ax.legend(loc='upper left')
# Add secondary axis for volume in price_ax
volume_ax2 = price_ax.twinx()
volume_ax2.bar(stock_data.index, stock_data['Volume'], color='gray', alpha=0.3, label='Volume')
volume_ax2.plot(stock_data.index, stock_data['Avg_Volume_20'], color='purple', label='20-Day Avg Volume', alpha=0.3)
volume_ax2.set_ylabel('Volume')
volume_ax2.legend(loc='lower left')
# Plot volume ratio in volume_ax
volume_ax.plot(stock_data.index, stock_data['Volume_Index'], label='Volume to MA Ratio', color='green')
volume_ax.axhline(y=dynamic_threshold, color='red', linestyle='--', label=f'{percentile_level*100:.0f}% Threshold')
volume_ax.set_title(f'{stock_symbol} Volume Ratio Over Time')
volume_ax.set_ylabel('Volume Ratio')
volume_ax.legend(loc='upper left')
# Plot histogram of volume ratio in hist_ax
hist_ax.hist(stock_data['Volume_Index'], bins=50, color='green', alpha=0.7, label='Distribution of Volume Ratios')
hist_ax.axvline(x=dynamic_threshold, color='red', linestyle='--', label=f'{percentile_level*100:.0f}% Threshold')
hist_ax.set_title(f'{stock_symbol} Volume Ratio Histogram')
hist_ax.set_xlabel('Volume Ratio')
hist_ax.set_ylabel('Frequency')
hist_ax.legend(loc='upper left')
# Adjust the layout for better clarity and show the plots
plt.tight_layout()
plt.show()
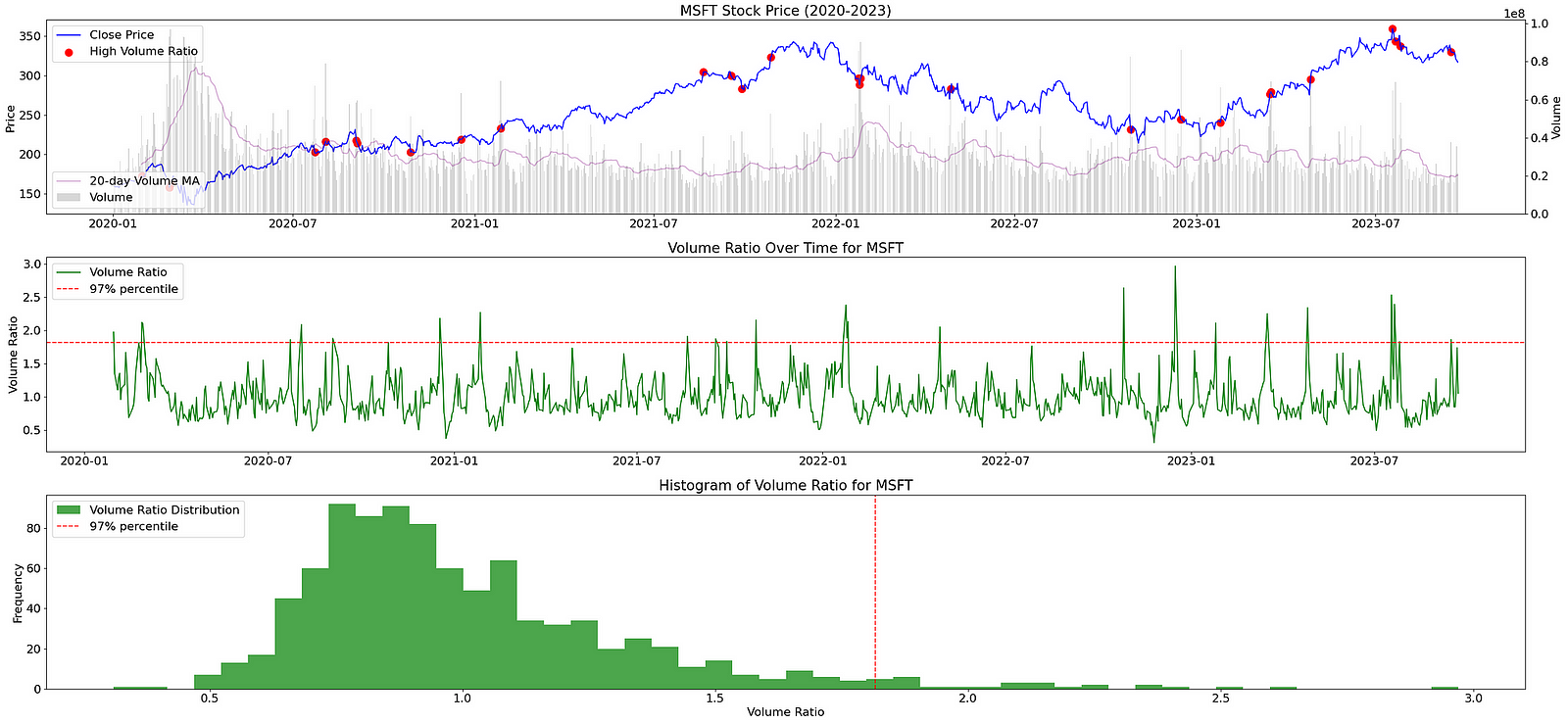
Figure 1. Visualizing Microsoft’s Stock Dynamics (2020–2023): A Comprehensive View of Price Evolution, Key Volume Ratios, and Their Distribution. The highlighted points indicate days where the volume ratio exceeded the 97th percentile, suggesting potential significant shifts in the market.
3. Analyzing Results and Deriving Insights
3.1 Reading the Plots
The generated plots provide visual tools designed to support informed decision-making. Proper interpretation is crucial to extract the most insightful conclusions:
Price Evolution Plot: The main line shows the stock’s closing price throughout the specified period. Red dots are marked at points when the volume ratio exceeds the predefined threshold, indicating notable trading days that could signal key market changes or shifts.
Volume Ratio Over Time: This plot visualizes the volume ratio with the green line, highlighting significant trading days. The red dashed line represents the threshold; when the green line crosses this line, it signals heightened trading activity.
Histogram of Volume Ratio: This distribution plot shows how trading volume ratios are spread across all days. The majority of the days will fall around the mean, but outliers on the higher end (especially those past the red dashed line) are the ones to focus on, as they might indicate abnormal market activity.
3.2 Strategic Implications
High volume ratio days, identified in the analysis, often precede or coincide with substantial price movements. These days signal significant market activity that could be influenced by factors such as news releases, earnings reports, or broader macroeconomic developments.
Tactical Adjustments: Traders may want to adjust their positions based on these insights. For example, an unusually high volume ratio paired with an upward trend could be interpreted as a strong buying signal.
Comprehensive Analysis: While the volume ratio is an insightful metric, it is essential to complement it with other technical and fundamental indicators. Tools like moving averages, the Relative Strength Index (RSI), or other fundamental analyses can offer a broader and more accurate market picture, resulting in a more robust trading strategy.
4. Conclusion
In this analysis, we explored the relevance of the volume ratio in stock trading, its calculation, and the development of a Python-based visualization tool to aid in decision-making. These visualizations highlight potential market-moving days that could signal important shifts.
For traders and researchers, integrating volume ratios into their analysis can provide valuable insights for decision-making. However, as with any approach in the stock market, caution is key. Given the volatility and the multitude of market influences, ongoing education, flexibility, and adaptability remain vital for success.